Spring Boot Security part 1
- Nguezang Arsene
- May 19, 2019
- 3 min read
Spring makes it easy to create Java enterprise applications. It provides everything you need to embrace the Java language in an enterprise environment, with support for Groovy and Kotlin as alternative languages on the JVM, and with the flexibility to create many kinds of architectures depending on an application’s needs. As of Spring Framework 5.1, Spring requires JDK 8+ (Java SE 8+) and provides out-of-the-box support for JDK 11 LTS.
A number of projects are associated with the spring framework and include the following
Spring boot
Spring Framework
Spring Web Services
Spring Security and many more
Our interest is how to incorporate the features of spring security in a spring boot web project.
We will create a simple informative web page and add Spring Security to it. To do this we will go through the following.
Requirements
Jdk 8 and above
An IDE (I will use IntelliJ IDEA but you can use any other that is java compatible)
Problem Statement
To implement Spring Security on a simple spring web project
Approach
step1. Create a new Spring Initializer project on Intellij

Next give your project a GroupId and an ArtifactId

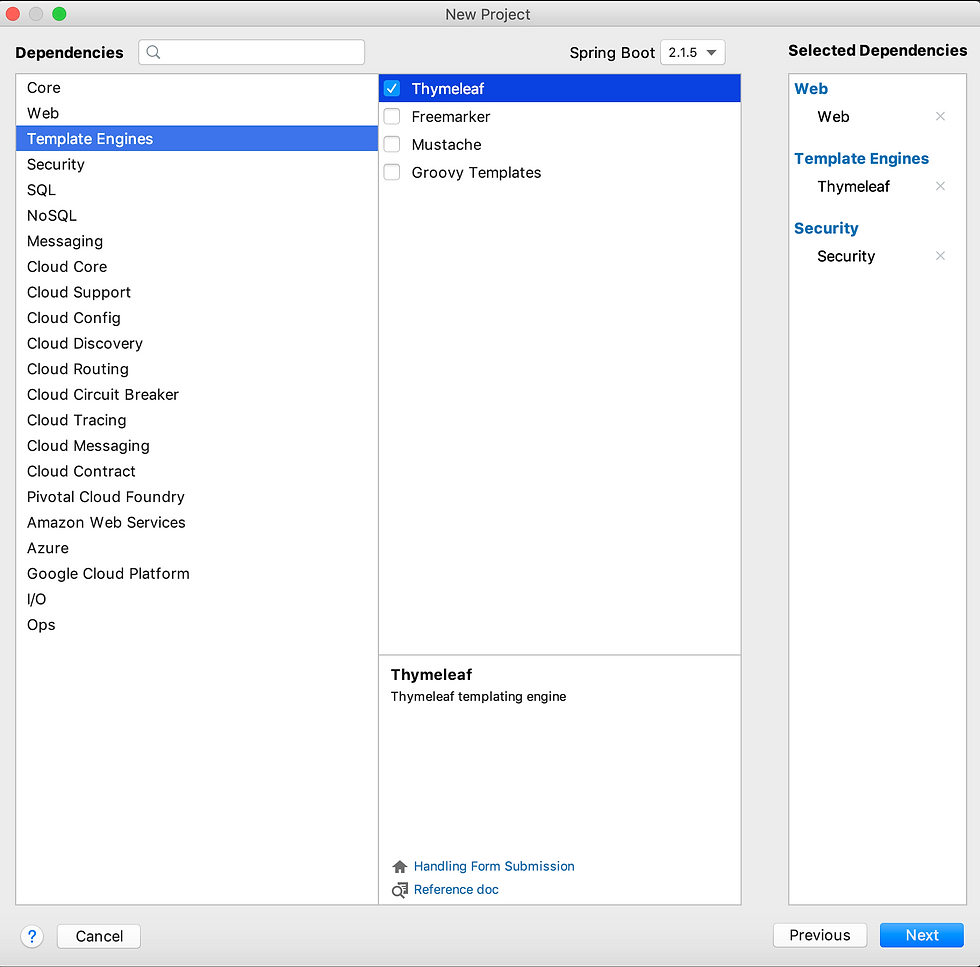
Add dependencies to your project. For purposes of simplicity, we add web, thymeleaf and spring security . We give our project a name (SimpleSpringSecurityTutorial).

Step 2. Verify that all the dependencies associated with security and thymeleaf are in the pom.xml file
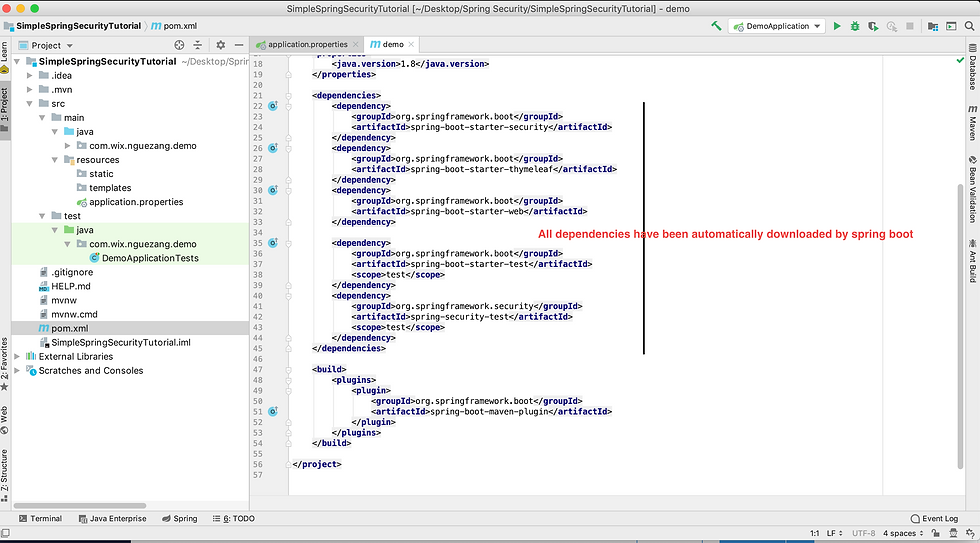
Step 3: We will create a simple index page for our application
<!DOCTYPE html> <html lang="en" xmlns:th="http://www.thymeleaf.org" xmlns:layout="http://www.ultraq.net.nz/thymeleaf/layout" layout:decorate="~ome/{#}"> <head> <link rel="stylesheet" type="text/css" th:href="@{/css/home/index.css}"/> <title>Home</title> </head> <body> <th:block layout:fragment="content"> <h3 style="color: lightskyblue;">Welcome to the Spring Security Demo</h3> <hr/> <div style="font-size: 1em"> <p>Lorem Ipsum is simply dummy text of the printing and typesetting industry. Lorem Ipsum has been the industry's standard dummy text ever since the 1500s, when an unknown printer took a galley of type and scrambled it to make a type specimen book. It has survived not only five centuries, but also the leap into electronic typesetting, remaining essentially unchanged. It was popularised in the 1960s with the release of Letraset sheets containing Lorem Ipsum passages, and more recently with desktop publishing software like Aldus PageMaker including versions of Lorem Ipsum.</p> </div> </th:block> <th:block layout:fragment="footer"></th:block> </body> </html>
Step 4:We create a simple controller class to handle this page
package com.wix.nguezang.demo.controller; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.GetMapping; /** * @author Nguezang Arsene */ @Controller public class HomeController { @GetMapping(value = {"", "/springsec"}) public String index() { return "home/home"; } @GetMapping(value = {"/springsec/home"}) public String home() { return "home/home"; } }
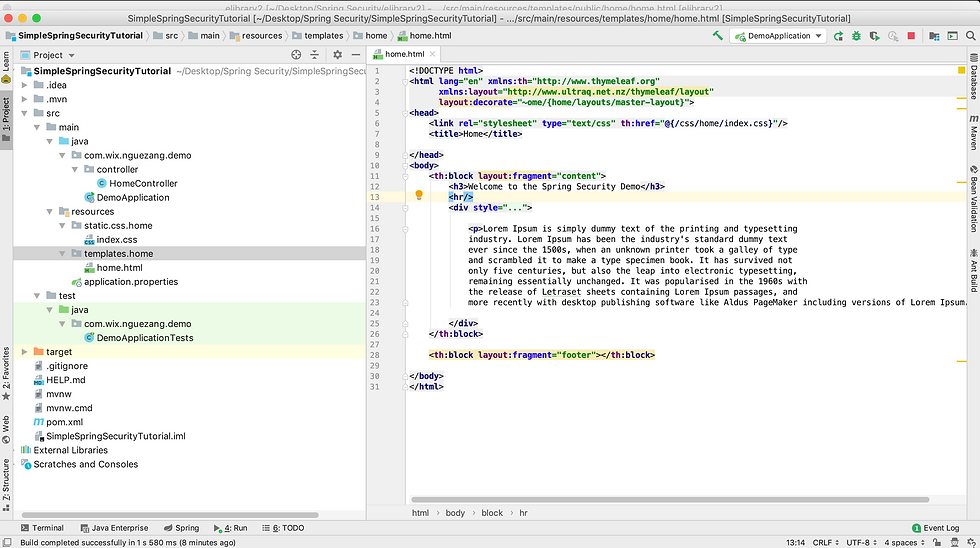
Step 5: We run the application

On the browser, when we go to the default port at localhost:8080 we have

You should be asking yourself why you are seeing a login page when we never coded one. That's the power of spring security. By adding spring security as one of our dependencies during project creation, spring automatically provides this login for us. The password for this is generated at runtime by the framework. The default username is user and the password can be copied from the console when the project starts. Failure to enter these will give you an error as shown below. You can get the dependency for spring security on the maven repository website
<dependency> <groupId>org.springframework.security</groupId> <artifactId>spring-security-test</artifactId> <scope>test</scope> </dependency>
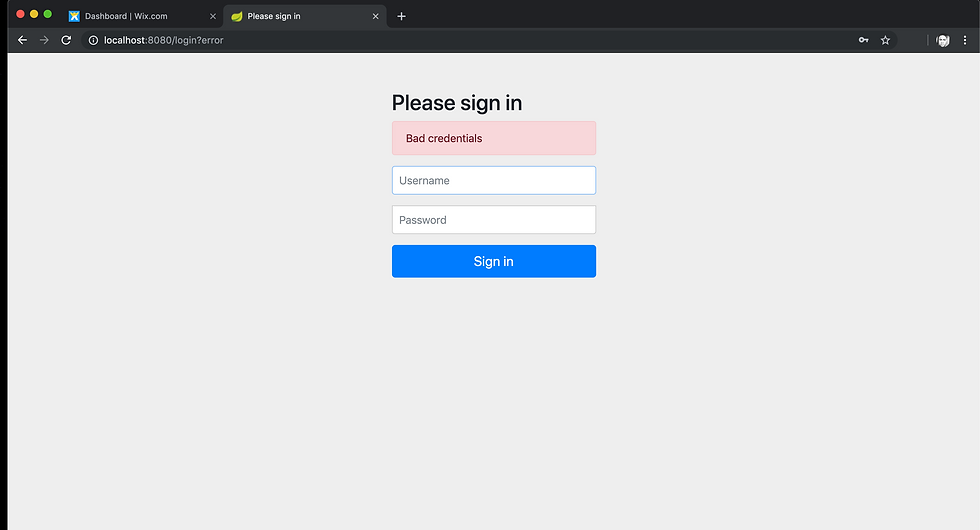
Now let's enter the right credentials and view the simple home page

Notes
Thymeleaf is a Java library. It is an XML/XHTML/HTML5 template engine that is able to apply a set of transformations to template files in order to display data and/or text produced by your applications.
Conclusion
This is a very basic web page that is secured by spring. This security can be customized for different project requirements. More on spring security here https://spring.io/projects/spring-security
Complete codes for this post can be found here https://github.com/nguezangarsene/BlogPostsCodes/blob/master/SimpleSpringSecurityTutorial.zip
Great Tutorial