Spring and the SpringMVC Framework: Lesson 1
- Nguezang Arsene
- Aug 2, 2019
- 3 min read
Spring Framework
A framework that supports the development of java applications. We configure different components to work together efficiently. With this, we are able to build applications from POJOs(Plain Old Java Objects). There is Little or no interference of the business logic into the framework.
Spring has a layered (N-tier) architecture made up of 3 main layers (tiers)
Presentation :Objects like the SpringMVC controllers are configured in the presentation context(tier)
Service Objects: Business specific objects exists in the business context (tier)
Data access objects exist in the persistence context(tier)
Spring MVC main features
Single Central Servlet: The FrontController or DispatcherServlet manages HTTP level request/response and delegate these to the appropriate interfaces
Separation of concerns
Views are plug and play : Views work perfectly when first used or connected without reconfiguration or adjustments by the user
Controllers are HTTP agnostic: No need to use the Servlet API, Spring's API is wrapped on top of this.
Spring MVC Major Interfaces
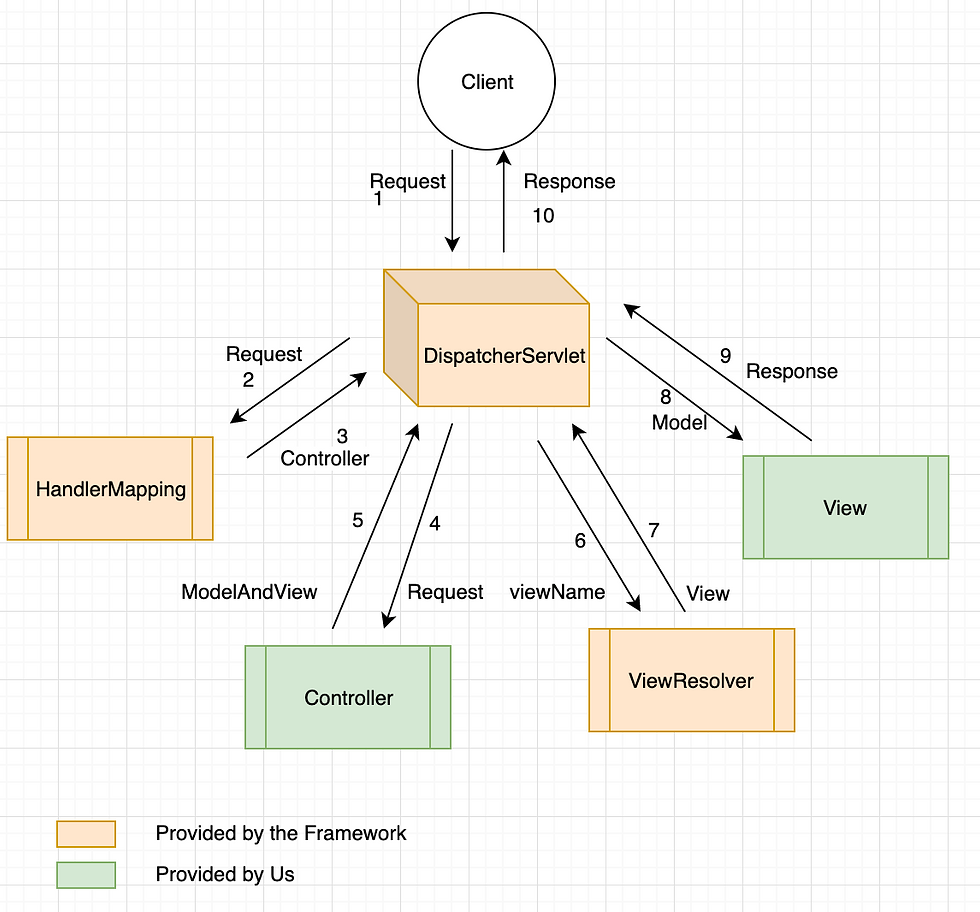
SpringMVC flow based on the above diagram
The DispatcherServlet first receives a request from the client
The DispatcherServlet consults the HandlerMapping and invokes the Controller associated with the request
The HandlerMapping returns the Controller that should handle this request
The Controller processes the request by calling the appropriate service methods
The Controller returns the ModelAndView object to the DispatcherServlet (The ModelAndView object contains the model data and the view name).
The DispatcherServlet sends the view name to the ViewResolver to find the actual View to invoke.
The view Resolver returns the view
The DispatcherServlet passes the model to the view to render the result
The model data is bound to the view, rendered and sent back to the DispatcherServlet
The DispatcherServlet returns this response back to the client that builds and displays this to the user.
Spring MVC Front Controller Configuration
Create a new Maven Project and in the web.xml file, add the following configurations in order to use the DispatcherServlet
In the pom.xml file, add the following dependencies
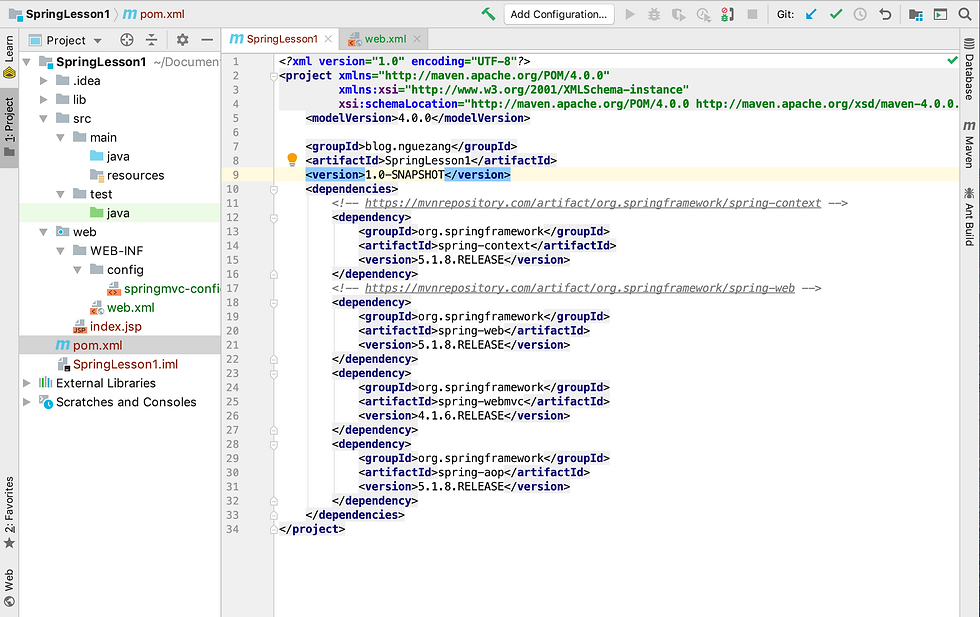
Then create a folder called config (directory) in WEB-INF, inside this folder, create a new xml file called springmvc-config.xml and add the following codes to it.

line 7 to 15
Spring’s dispatcher servlet is implemented by the class org.springframework.web.servlet.DispatcherServlet. The initialization parameter contextConfigLocation tells Spring where to load configuration files. The <load-on-startup> tag tells the servlet container to load this servlet upon start up with highest priority.
Line 17 to 20
Tells the container to route all requests to Spring’s dispatcher servlet. For example, the following URL will be processed by the dispatcher servlet:
http://localhost:8088/SpringLesson1/
JavaConfig Version
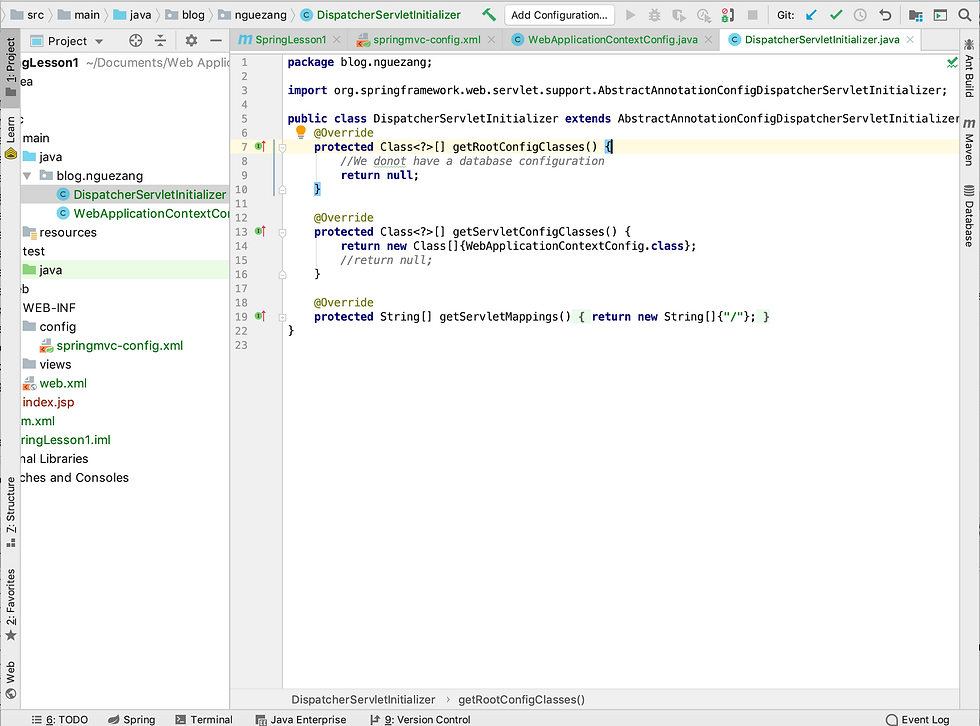
springmvc-config.xml

<context:component-scan base-package="pkg,pkg1.."/>
Scans defined packages to find and register @Component-annotated classes and activate basic annotations[e.g. @Autowired] - within the current application context.
<mvc:annotation-driven/>
Enables support for specific annotations [e.g. @RequestMapping, etc.] that are required for Spring MVC to dispatch requests to @Controllers. It is based on MVC XML namespace.
<tx:annotation-driven/>
Enables support for specific annotations that are required for Spring Transactions @Transactional. It is based on transaction XML namespace.
JavaConfig Version
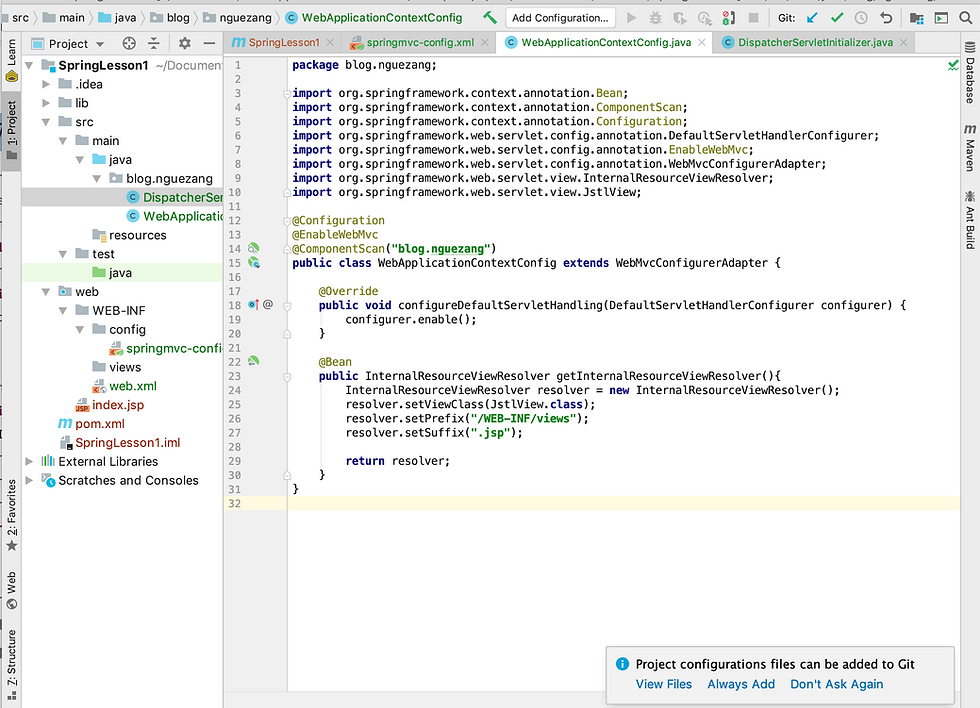
@Configuration This indicates that a class declares one or more @Bean methods. @EnableWebMvc imports some special Spring MVC configuration @ComponentScan("blog.nguezang") This specifies the base packages to scan for annotated components(beans)
Spring MVC Annotations

Here are some of the common annotations used in SpringMVC
@Component: It means that the Spring framework will autodetect these classes for dependency injection when annotation-based configuration and class path scanning is used.
@Repository - automatic translation of exceptions
@Controller – rich set of framework functionality
@Service – “home” of @Transactional
@RequestMapping
@ModelAttribute
@RequestParam
@SessionAttributes
More about the use of these annotations in the coming lessons
Data Binding
Automatically maps request parameters to domain objects
Simplifies code by removing repetitive tasks
Built-in Data Binding handles simple String to data type conversions
HTTP request parameters [String types] are converted to model object properties of varying data types.
Does NOT handle COMPLEX data types; that requires custom formatters
Does handle complex nested relationships
Inversion of Control (IOC)
Promotes loose coupling between classes and subsystems
Adds flexibility to the code for future changes
Classes are easier to unit test in isolation
IOC (Inversion of Control) is implemented using Dependency Injection (DI)
Dependency Injection (DI)
The Container injects dependencies when it creates the bean Dependency Injection Types Examples
1. Property based(byType)
@Autowired
ProductService productService;
2. Setter based(byName)
ProductService productService;
@Autowired
public void setProductService(ProductService productService){ this.productService = productService;
}
3. Constructor based:
ProductService productService;
@Autowired
public ProductController(ProductService productService) { this.productService = productService;
}
Note: Best practice is to use Constructor based dependency Injection because it automatically enforces the order and completeness of the instantiated objects. Furthermore, when the last object is instantiated the wiring phase of your application is completed.
Notes and References
Comments